Fusion360 APIの文字列入力ダイアログ
2021/10/10 categories:Fusion360| tags:Fusion360|Fusion360 API|Python|
Fusion360 APIで文字列入力のダイアログを表示してみました。
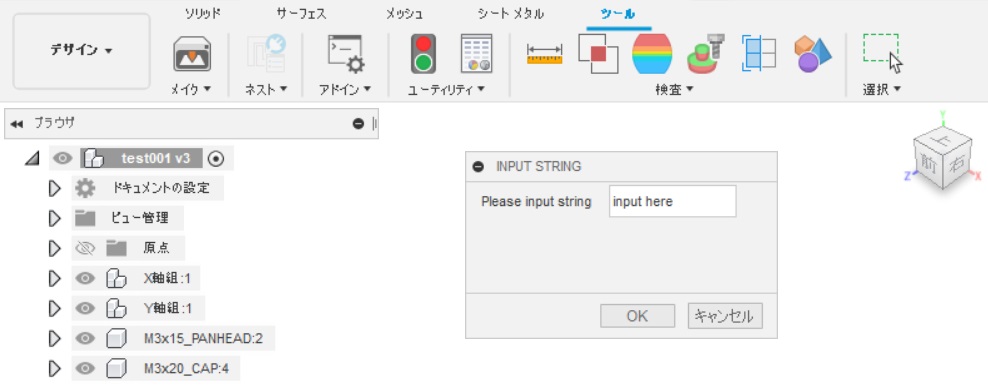
ハンドラーの作成
下記2つのクラスを継承して、コマンド作成、コマンドイベント作成、コマンド破棄のクラスを作成しました。
- adsk.core.CommandEventHandler
- adsk.core.CommandCreatedEventHandler
コマンド作成クラス
このクラスでは以下の処理を行います。
- コマンドに実行イベントを追加
- コマンドに破棄イベントを追加
- ハンドラーのリストにイベントを追加
- コマンドに文字列入力のUIを追加
class PartsCounterCommandCreatedHandler(adsk.core.CommandCreatedEventHandler):
def notify(self, args):
try:
cmd = args.command
cmd.isRepeatable = False
onExecute = PartsCounterCommandExcuteHandler()
cmd.execute.add(onExecute)
onDestroy = PartsCounterCommandDestroyHandler()
cmd.destroy.add(onDestroy)
handlers.append(onExecute)
handlers.append(onDestroy)
inputs = cmd.commandInputs
inputs.addStringValueInput('Input string', 'Please input string', 'input here')
except:
if ui:
ui.messageBox('Failed:\n{}'.format(traceback.format_exc()))
コマンド破棄クラス
ダイアログのキャンセルをクリックしたときにコマンドが終了するようにadsk.terminate()を実行するクラスを作成しました。
class PartsCounterCommandDestroyHandler(adsk.core.CommandEventHandler):
def notify(self, args):
try:
adsk.terminate()
except:
if ui:
ui.messageBox('Failed:\n{}'.format(traceback.format_exc()))
コマンド実行クラス
ダイアログのOKをクリックしたときに、コマンドを終了して、入力した文字列を表示するクラスを作成しました。args.firingEvent.senderでイベントを実行したオブジェクトを取得出来て、そのオブジェクトのcommandInputsから値を取得します。取得した値はui.messageBox()で表示します。
class PartsCounterCommandExcuteHandler(adsk.core.CommandEventHandler):
def notify(self, args):
try:
adsk.terminate()
command = args.firingEvent.sender
input_value = command.commandInputs[0].value
ui.messageBox(input_value)
except:
if ui:
ui.messageBox('Failed:\n{}'.format(traceback.format_exc()))
実行結果
スクリプトを実行すると以下のようにダイアログが表示されます。
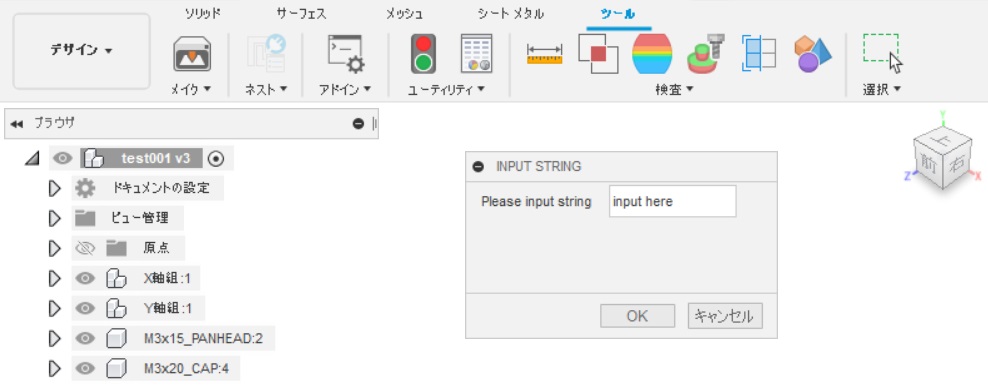
上のダイアログで文字列を入力してOKをクリックすると以下のようにメッセージボックスが表示されます。
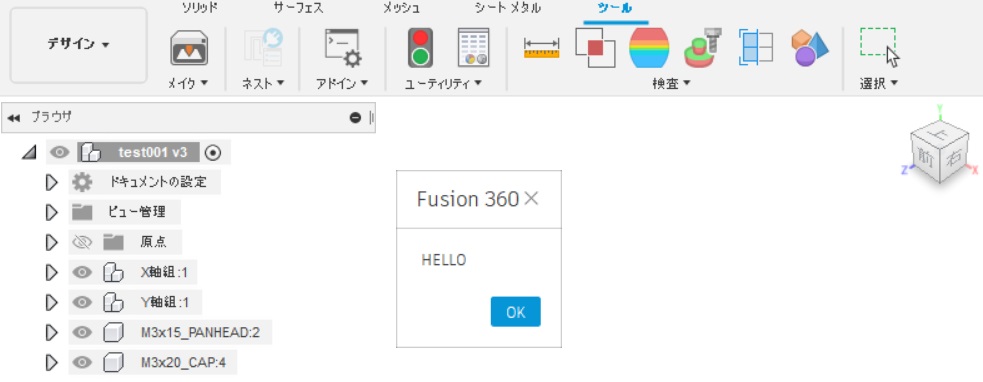
ソースコード
import adsk.core, adsk.fusion, adsk.cam, traceback
handlers = []
app = adsk.core.Application.get()
if app:
ui = app.userInterface
class PartsCounterCommandExcuteHandler(adsk.core.CommandEventHandler):
def notify(self, args):
try:
adsk.terminate()
command = args.firingEvent.sender
input_value = command.commandInputs[0].value
ui.messageBox(input_value)
except:
if ui:
ui.messageBox('Failed:\n{}'.format(traceback.format_exc()))
class PartsCounterCommandDestroyHandler(adsk.core.CommandEventHandler):
def notify(self, args):
try:
adsk.terminate()
except:
if ui:
ui.messageBox('Failed:\n{}'.format(traceback.format_exc()))
class PartsCounterCommandCreatedHandler(adsk.core.CommandCreatedEventHandler):
def notify(self, args):
try:
cmd = args.command
cmd.isRepeatable = False
onExecute = PartsCounterCommandExcuteHandler()
cmd.execute.add(onExecute)
onDestroy = PartsCounterCommandDestroyHandler()
cmd.destroy.add(onDestroy)
handlers.append(onExecute)
handlers.append(onDestroy)
inputs = cmd.commandInputs
inputs.addStringValueInput('Input string', 'Please input string', 'input here')
except:
if ui:
ui.messageBox('Failed:\n{}'.format(traceback.format_exc()))
def run(context):
try:
product = app.activeProduct
design = adsk.fusion.Design.cast(product)
if not design:
ui.messageBox('It is not supported in current workspace, please change to MODEL workspace and try again.')
return
commandDefinitions = ui.commandDefinitions
cmdDef = commandDefinitions.itemById('Input string')
if not cmdDef:
cmdDef = commandDefinitions.addButtonDefinition('Input string', 'Input string', 'Parts count', './resources')
onCommandCreated = PartsCounterCommandCreatedHandler()
cmdDef.commandCreated.add(onCommandCreated)
handlers.append(onCommandCreated)
inputs = adsk.core.NamedValues.create()
cmdDef.execute(inputs)
adsk.autoTerminate(False)
except:
if ui:
ui.messageBox('Failed:\n{}'.format(traceback.format_exc()))